Selenium Automation Troubleshooting Tips
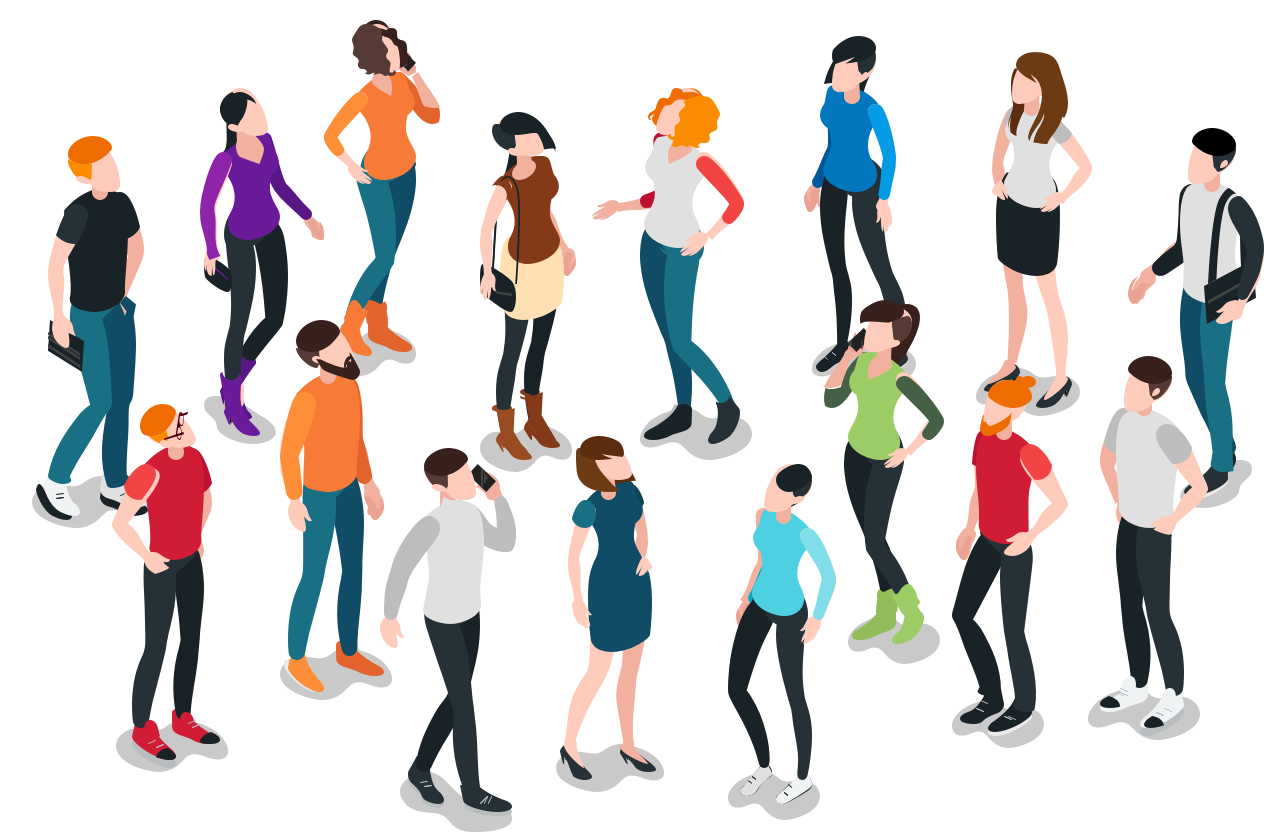
If same element is available on different pages with same attributes and they do not have any unique attributes which can differentiate them from one another then driver.findElement() may not work if this element has been loaded several times in browser during one test on different pages. Selenium returns first match found using locator and in such situations old element may be available in cache which is not displayed but is available to Selenium and hence selenium will click old element from cache and go ahead to next step. In such cases error will not be thrown on this step but on steps ahead which makes it hard to identify issue.
Consider a web application with 3 different tabs on a web page, each having a drop down “View By” which has two list options : 1) Name 2) Component
Given below is HTML for “Name” option from two different tabs which are exactly same except their ids which also can not be used to differentiate between the two tabs either since id here is similar dynamic pattern
In such cases a simple locator like xpath=”//td[text()=’Name’]” can be used to find all elements and then work with last element found since currently loaded element will be the last in list of elements Selenium found using the locator and old elements present in cache wont cause an issue. Example 1 shows one way to find last element matching a locator.
Example 1. Working with multiple occurrence of similar elements
/** Return last web element found by a locator */
public WebElement getLastWebElement(String locator){
log.info(“Find similar web elements and return Last webElement identified by “+ locator);
List
if(els!=null)
return ListElements.get(ListElements.size()-1);
else
return null;
}
If locator is correct and your test case uses similar element multiple times in same test from different pages, it could again be due to old element being present in cache hence work with last element found using locator as shown in Example 1.
When an element is present on currently loaded page but not visible on screen and driver.findElement().click() is not able to click on element. Or in case of making selection in drop down or combo box, if option is not visible on screen. Or when an element on a child dialog can not be seen and is hidden, then try any of the below Example 2a, 2b or 2c to bring element in focus before working with element.
Example 2a. Work with elements which are not seen on screen
WebElement element = driver.findElement(By.cssSelector(“myselector”));
Actions actions = new Actions(driver);
actions.moveToElement(element);
Example 2b. Work with elements which are not seen on screen
First scroll on current screen/dialog using below. Here example shows vertical scroll, similar can be applied to horizontal scroll if required.
WebElement element = driver.findElement(By.cssSelector(“myselector”));
((JavascriptExecutor)driver).executeScript(“window.scrollBy(0,”+value+”)”, “”);
Example 2c. Work with elements which are not seen on screen
WebElement element = driver.findElement(By.cssSelector(“myselector”));
((JavascriptExecutor) driver).executeScript(“arguments[0].scrollIntoView(true);”, element);
Some times click on locator does not work when clicked on a menu item, in this case one needs to use send key method to send return key instead of using click.
Example 3. Use Actions.sendKeys() to work with menu items
For example test steps require user to click on File menu and the it’s menu item “Move”. But automation keeps clicking next menu to File instead of clicking menu item of File menu “Move”. To fix such issue use sendKeys() method to use combination of arrow and return keys.
Driver.findElement(By.id(File)).click();
Actions action= new Actions(driver);
action.sendKeys(Keys.RETURN).perform();
action.sendKeys(Keys.DOWN).perform();
action.sendKeys(Keys.RETURN).perform();
The code in Example 3 does the same as “File” followed by click on “Move”.
To perform right-click on an element, use the interaction class Action, two options are shown in Example 4a and 4b.
Example 4a. Interaction class for right click
Actions action= new Actions(driver);
action.contextClick(driver.findElement(By.xpath(“my xpath locator”))).clickAndHold().perform();
Example 4b. Interaction class for right click
Actions action= new Actions(driver);
action.contextClick(driver.findElement(By.xpath(“my xpath locator”))).build().perform();
Catch all possible exceptions in task classes and exit gracefully post exception by calling tear down.
If using TestNG framework, use tear down method with alwaysRun set to true as shown in Example 6 to ensure tests cleans up after itself irrespective of test result.
Example 6. Use tear down with alwaysRun set to true
/** Tear down */
@AfterClass(alwaysRun=true)
public void tearDown(){
// Cleanup code here
}
Even after following good practices and using great care when creating locators, it is not unlikely for tests to fail when run multiple times. Therefore it is important to run a test multiple times so it uncover issues that might not have been encountered when run fewer times. It is recommended to practice below test approach
Run each test a minimum of 15-20 times.
Run tests in multiple test environments.
Run tests in non development environment. This helps uncover browser version or timing issues.
Run multiple tests together regularly to ensure the tests run together and there are no residual from a test which adversely affects other tests.
Yes this is good!
This is what i want! thanks!